A three minute introduction into shorthand variable assignment
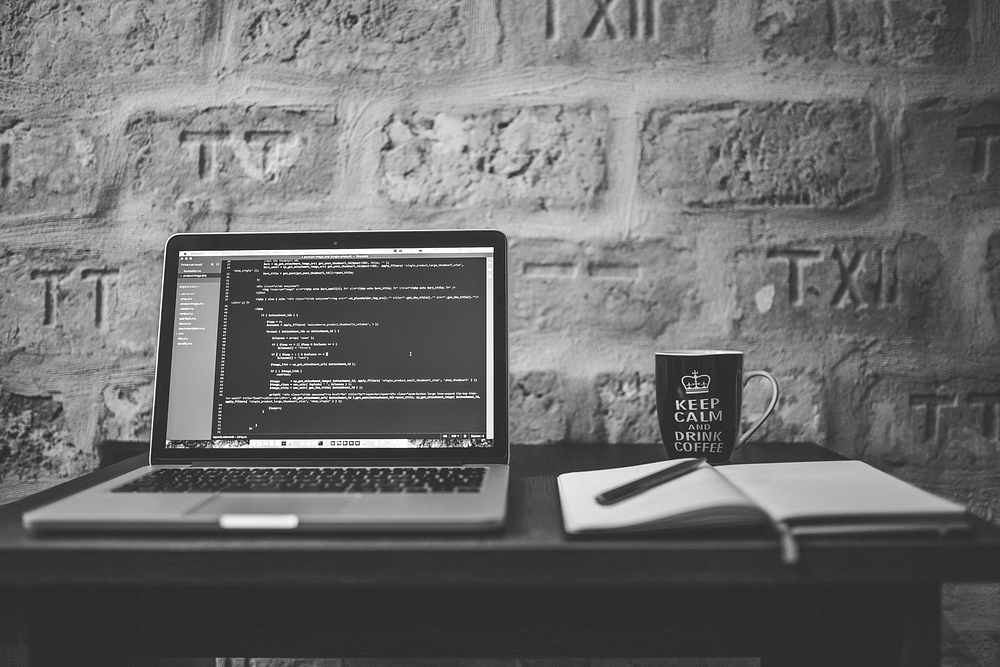
This article will take a (very) quick look at shorthand variable assignment in JavaScript.
Assigning Variables to Other Variables
As you’re probably aware, you can assign values to variables separately, like this:
var a = 1; var b = 1; var c = 1;
However, if all variables are being assigned equal values, you can shorthand and assign the variables like this:
var a = b = c = 1;
The assignment operator
=
in JavaScript has right-to-left associativity. This means that it works from the right of the line, to the left of the line. In this example, here is the order of operation:- 1 — First,
c
is set to1
. - 2 — Next,
b
is set equal toc
which is already equal to1
. Therefor,b
is set to1
. - 3 — Finally,
a
is set equal tob
which is already equal to1
. Therefor,a
is set to1
.
As you can now see, the shorthand above results in
a
, b
, and c
all being set to 1
.
However, this is not a recommended way to assign variables. That’s because in the shorthand variable assignment shown above, we actually never end up declaring variables
b
or c
. Because of this, b
and c
wont be locally scoped to the current block of code. Both variables b
and c
will instead be globally scoped and end up polluting the global namespace.Using Commas When Assigning Variables
Lets look at a new example. Consider the following variable declarations and assignments:
var d = 2; var e = 3; var f = 4;
We can shorthand this code using commas:
var d = 2, e = 3, f = 4;
As you see, we are separating each variable assignment with a comma which allows us to assign different values to each variable.
For ease of reading, most coders who prefer using the comma method will structure their variable assignments like this:
var d = 2, e = 3, f = 4;
Best of all, in the shorthand variable assignment shown above, we are declaring all three variables:
d
, e
, and f
. Because of this, all variables will be locally scoped and we’re able to avoid any scoping problems.Want to Learn More Shorthands?
Check out my other articles on shorthand coding techniques in JavaScript:
- JavaScript — The Conditional (Ternary) Operator Explained
- JavaScript — Short Circuit Conditionals
- JavaScript — Short Circuit Evaluation
Closing Notes:
Thanks for reading! If you’re ready to finally learn Web Development, check out: The Ultimate Guide to Learning Full Stack Web Development in 6 months.
If you’re working towards becoming a better JavaScript Developer, check out: Ace Your Javascript Interview — Learn Algorithms + Data Structures.
No comments:
Write comments